Top REST API URL naming convention standards
There is no sanctioning body or open source linter that can verify if a RESTful API conforms and complies with all applicable REST API naming conventions and best practices.
However, REST API developers should follow certain industry-wide accepted naming conventions and guidelines.
Guidelines for naming RESTful APIs
Some of these best practices for naming RESTful APIs are tied tightly to the rules about how best to format URLs for HTTP. Others stem from the technical guidance in Roy Fielding's dissertation on representational state transfer.
Regardless of their inspiration, developers should follow all the REST naming conventions to create an API that is easy to follow and work with and complies with the standards expected of a RESTful resource.
REST API URL naming conventions
The following list represents the standard set of REST API naming conventions and best practices that software architects should follow when they develop and design RESTful APIs:
- Only use lowercase letters in RESTful API URLs.
- For white space, use kebab-case, not snake_case or spaces.
- Build URIs with nouns, not verbs.
- Use the appropriate HTTP methods to perform an operation.
- REST API calls that return a collection should be plural.
- A URL that returns a unique result should be singular.
- Don't include file extensions.
- Use headers to keep URIs clean.
- Don't identify Create, Read, Update and Delete (CRUD) operations in the URL.
- Structure URIs loosely on your data model's hierarchy.
- Use query parameters for filtering and search.
- Don't expose the internal workings of your architecture.
- Make URLs short, intuitive and readable.
- Protect yourself against SQL injection.
- Include the REST API version at the base of the URI.
RESTful URLs should be lower case
Never waste time figuring out if a RESTful API call failed because the URL included an upper-case letter instead of a lowercase one.
RESTful URLs should only contain lower-case letters. That's a RESTful API naming convention nobody should ever violate.
Good and bad RESTful API URL examples in the following:
- https://www.example.com/v1/sample/uri #Good.
- https://www.example.com/v1/SampleURI #Bad.
Use kebab-case, not snake_case
Sometimes it's easier to read a URL if it has a whitespace-heavy separator. A dash between words is fine if it makes the URL more readable.
However, a REST API URL should never contain a space. Never use snake_case in a RESTful API, as the underscore can easily be misread as an empty space. Under no circumstance should you use SCREAMING_SNAKE_CASE, there's never any excuse for that.
Examples include the following:
- https://www.example.com/v1/this-is-okay
- https://www.example.com/v1/THIS_IS_NOT_OKAY
Use nouns, not verbs
The R in URI stands for "resource," and resources are nouns.
The URL for a RESTful API should point at resources that can be manipulated. Resources are things, which are nouns. Only include nouns in your URI.
Examples include the following:
- https://www.example.com/v1/players/gretzky #Good.
- https://www.example.com/v1/players/get-gretzky #Bad.
Use HTTP methods over verbs
The HTTP protocol provides numerous methods to identify the types of operations a RESTful API call intends to perform on a resource.
Common HTTP methods, and the operations to which they commonly map, include the following:
- GET to retrieve data about a resource.
- DELETE to remove a resource.
- PUT to replace or create a resource.
- PATCH to update a resource.
- POST to manipulate a resource without idempotence.
Don't ever let a verb sneak into your URIs. If an operation must be performed, use the appropriate HTTP method.
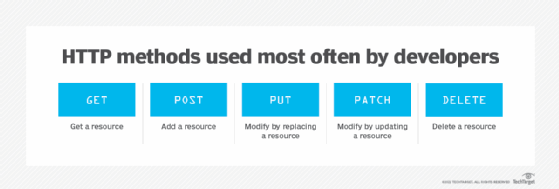
Collections are plural
When a RESTful API call returns a collection of resources, the URI should be plural. Similarly, if the expected result is singular, so should be the URI:
- https://www.example.com/v1/teams #Acts on a collection of teams.
- https://www.example.com/v1/teams/{identifier} #Acts on a single team.
Do not include file extensions
If the expectation is that the client opens a URL with a specific program, such as a web browser or PDF viewer, then it's acceptable to end a URL with a file extension.
Otherwise, per REST API naming convention best practice, do not include a file extension in a URL. Use a MIME-type header to inform the client about the type of data that is passed across the network.
Use headers to keep URIs clean
Developers have the option to transfer data across over the HTTP protocol using hidden headers or visible URL parameters.
It's a REST API best practice to pass supplemental data, such as access tokens or session IDs, as headers to keep the URL relatively clean. A URL gets busy enough without an additional 128-character hexadecimal code.
Do not identify CRUD operations in the URL
This ties back to the REST API best practice of relying on HTTP methods for resource operations. However, the anti-pattern of including CRUD operations, such as Read, Delete, Update or Create, in a URL is so common that it deserves its own spotlight.
Do not include CRUD operations in the URI. The URI is no place for verbs. Always use HTTP headers to indicate the intent of an operation you wish to perform.
Bad RESTful API URL examples include the following:
- https://www.example.com/v1/teams/blue-jays/delete #Bad.
- https://www.example.com/v1/teams/blue-jays/retrieve #Bad.
Structure REST APIs in a hierarchical manner
RESTful APIs should be logical and predictable, with increasingly specific relationships between resources as the URI reads from left to right.
Here's a simple example of what a mapping API URL might look like https://www.example.com/v1/map/earth/north-america/usa/boston.
You can see that URL shows a logical relationship between resources, and more specific relationships between resources as the URL extends.
Use query parameters to simplify RESTful URI structures
Imagine your mapping app offers directions in English and French. Where would the language code best fit in the URL?
Which of the following do you think is most in line with standard REST API naming convention best practices?
- https://www.example.com/v1/fr/map/earth/north-america/usa/boston
- https://www.example.com/v1/map/earth/north-america/usa/boston/fr/
- https://www.example.com/v1/map/fr/earth/north-america/usa/boston
Unfortunately, none of these approaches is perfect. However, a common anti-pattern for solution architects is to force every property of a resource into a URI hierarchy.
Quite often, search parameters are a better way to allow clients to interact with your RESTful APIs. Consider the following as a better approach to RESTful URI design:
- https://www.example.com/v1/map/fr/earth/north-america/usa/boston?lang=fr
The benefits of the query parameter approach to naming RESTful APIs are that it avoids forcing an unnatural hierarchy upon your implementation and enables developers to easily add new query parameters in the future.

Don't expose internal IDs in your REST APIs
Many organizations expose their database details through their REST APIs, including the ability to search for records based on a database record's primary key or unique identifier. This creates a situation in which hackers simply type in the next number in a sequence to view other records in your database, which can lead to major privacy and security penetrations.
Don't ever expose the internal workings of your system through a public REST API.
Make URIs short, intuitive and readable
Don't build your RESTful API as if you are a contestant on the Wheel of Fortune, spinning for a chance to select a letter but pay $500 for a vowel.
An important REST API naming convention best practice is to make URIs short, but not so short that they are illegible and unreadable. Intuitive and readable RESTful URIs are key. Don't abbreviate words, strip out vowels or intentionally misspell a resource name just to trim the URI by a letter of two.
Here are good and bad examples of REST API URLs:
- https://www.example.com/v1/tm/oilers/plyrs/gretzky #Bad.
- https://www.example.com/v1/team/oilers/players/gretzky #Better.
Protect yourself against SQL injection
Avoid using URI parameters that are shared with the SQL standard. You do not want your URIs to accidentally become prone to SQL injection attacks.
Any URL that sends data to the server as part of an HTTP request, especially if handling the request involves a database interaction, should be cleansed to protect against SQL injection attacks. Request parameters that use SQL keywords such as the CRUD operations complicate the data cleansing process, so avoid these terms.
Furthermore, never directly insert data obtained from a RESTful URL into a database query without proper validation. Always protect your backend from potential SQL injection attacks that can stem from bad actors manipulating the front end.
Explicitly version your APIs
REST API versioning was once a hotly debated topic, but the community has agreed upon the need to include the API version at the base of the URI.
Organizations often overlook this when they develop their very first API, but they shouldn't. Include the API version at the root of the URI and you'll avoid future problems when you need to migrate users to an updated implementation.
An example is https://www.example.com/v2/map/earth/north-america/usa/boston.
Best practices for naming RESTful URLs
Software architects and developers have a great deal of flexibility to develop RESTful APIs. While nobody wants to limit the creativity of the dev team, they should follow these REST API naming conventions, guidelines and best practices to produce an API that is maximally readable, interoperable and immediately understandable.
Karan Adapala is a full-stack developer, app architect and cloud enthusiast with expertise in React, Node.js, TypeScript, Python, Docker, Terraform, Jenkins and Git.