Create a React Hello World program
If Node.js is already installed on your computer, you can create a runnable React Hello World app by simply issuing the following command:
npx create-react-app hello-world
Create and run a React app
From there, inside the hello-world folder that gets created, run a single npm start command to start your app and make it available on port 3000 of localhost:
cd hello-world
npm start
The React Hello World app
To turn the provided app into a React Hello World program, simply add the text "Hello World!" to the src/App.js file. The webpage will refresh automatically and display the updated React Hello World app:
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<p>
Hello World!
</p>
</header>
</div>
);
}
export default App;
Beyond Hello World in React
This React Hello World tutorial demonstrates how to get a basic program up and running, but it doesn't stop there.
Once your Hello World program works, it's a perfect time to explore how easy it is to incorporate advanced features into your React app, including the following:
- State management.
- Variable declarations.
- Method creation.
- Event handling.
- TypeScript syntax.
An advanced React Hello World tutorial
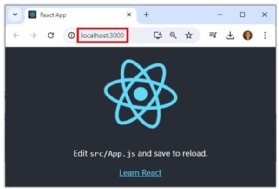
The whole point of creating a Hello World React program is to ensure your Node.js environment works, quickly examine the basic structure of a library or framework, and address any configuration issues before moving on to more advanced topics.
More broadly, however, React greatly simplifies the art of stateful website development. With a working Hello World app at your disposal, it would be a missed opportunity not to explore some of the additional features this popular library has to offer -- which is exactly what you'll learn about in this advanced React Hello World tutorial.
Cameron McKenzie has been a Java EE software engineer for 20 years. His current specialties include Agile development; DevOps; Spring; and container-based technologies such as Docker, Swarm and Kubernetes.