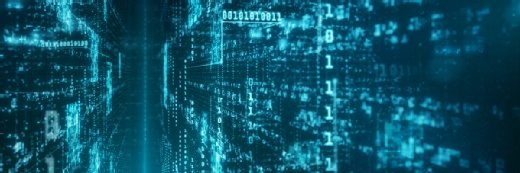
Getty Images
Swing vs. JavaFX: Compare Java GUI frameworks
Swing has been used with Java GUIs since 1998, but there are good reasons to adopt JavaFX instead. Here's how to know which to choose for your next Java project.
The key difference between Swing vs. JavaFX is that JavaFX is an actively maintained Java project that supports the development of modern, feature-rich GUI applications, while Swing is an older desktop app development framework that is currently feature-frozen and not recommended for new projects.
Desktop development in Java
Yes, developers can use Java to develop graphical user interfaces (GUIs). In fact, Java's ability to create cross-platform desktop apps was one of the language's biggest selling features when it was released in 1995.
However, as Java evolved and changed throughout the years, so did the desktop-rendering toolkits it supported, with the original Abstract Window Toolkit (AWT) giving way to Swing components which has now given way to JavaFX, the preferred choice for modern development.
5 reasons to choose JavaFX over Swing
While Swing and AWT both played important roles in the history of GUI development, both projects have their feature lists frozen, and their only active maintenance is for patches and bug fixes. In contrast, JavaFX is actively maintained and provides a variety of modern features that Swing and AWT simply don't support, including the following:
- A modern graphics engine.
- Support for modern web technology such as CSS.
- FXML support for templates and page design.
- Scene graph architecture.
- Multimedia and web integration.
Modern graphics engine
JavaFX uses a rendering pipeline as its graphics engine, called Prism. It supports hardware-accelerated graphics depending on the platform on which it runs, including OpenGL for Mac and Linux or Direct3D for Windows. A software rendering based on CPU rendering techniques is available for cases when no hardware acceleration is available. This provides superior performance and smooth animations in almost all cases.
CSS styling
Unlike Swing, you can use CSS to style your JavaFX interfaces. This makes it very easy to adapt the default look and feel to match, for instance, your corporate identity. Libraries such as AtlantaFX and JMetro add themes to an application to consistently adjust the styling across all components.
FXML support
When developers create a user interface with JavaFX, they can follow two different approaches:
- Code-only. All components are constructed with code and added to a layout component.
- FXML. The layout is created in an XML-based file with bindings to the code to handle, e.g., button presses.
The FXML approach results in a clearer separation between the design of the user interface and the application logic. Scene Builder is a WYSIWYG editor that helps developers create such FXML files. This approach is especially handy for development teams where different people create the layout and logic.
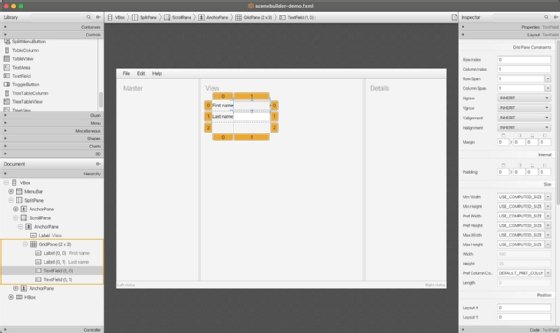
Scene graph architecture
Every visual element in JavaFX extends from a node and is represented in a treelike hierarchical structure. An element can either be a container (Group, Pane, HBox, etc.) or an individual component (Button, Label, etc.), with the Scene as the top-level container. Using this tree structure, a transformation or effect applied to the parent is also applied to any children. Because of this approach, the rendering pipeline could be optimized and the graphics hardware-accelerated.
Multimedia and web integration
The JavaFX framework contains components with built-in support for web views, media playback and 3D graphics. With these, developers can expand far beyond what was possible with traditional UI frameworks such as Swing and AWT.
The role of Swing and Java's AWT
Included as part of the original 1995 JDK release, AWT was the first library to support GUI development in Java.
While functional and easy to use, AWT had its drawbacks. Components rendered slowly, there was a disappointingly limited number of prebuilt components, and the ones that were provided were not easily extended. To address these complaints, the Swing components, and the Java Foundation Classes (JFC) that support them, were added to 1998's JDK 1.2 release.
The Swing components, and the framework Swing provided for GUI-based application development in Java, were well received by the community which used the technology to develop a variety of enterprise-grade desktop applications, including the popular IDE IntelliJ IDEA.
A minimal Swing application looks as follows:
import javax.swing.*;
/**
* You can execute this example with java:
* $ java HelloWorldSwing.java
*/
public class HelloWorldSwing {
public static void main(String[] args) {
JFrame frame = new JFrame("Hello Swing World!");
frame.setSize(300, 200);
// Create a label with the hello world message
JLabel label = new JLabel("This is a label", SwingConstants.CENTER);
frame.add(label);
// Make the frame visible
frame.setVisible(true);
}
}
The future of Swing and AWT
To maintain backward compatibility with projects that use them, both Swing and the legacy AWT components continue to be packaged with modern releases of the JDK. However, neither project is actively maintained; Swing now exists in "maintenance mode" where it only receives bug fixes and security updates but no new features.
When update 6 of Java SE 7 was released in June 2012, Oracle made it clear that JavaFX would replace Swing as the standard UI library for Java.
What is JavaFX?
JavaFX had a bit of a winding path.
It was first released in 2008, called JavaFX Script, and focused on desktop applications and web browsers. It was originally designed as a scripting language, but with the 2011 version 2 release it transitioned into a full-blown Java library that was distributed as part of the JDK. In 2018, with the release of Java 11, JavaFX morphed again into OpenJFX, a separate OpenJDK project on GitHub.
The module system introduced in Java 8 also fully embraced JavaFX. This resulted in a more granular dependency structure that enabled developers to integrate only those parts of it (controls, graphics, web, FXML, etc.) required for an application.
OpenJFX is no longer part of OpenJDK, but you can still get JDK installers that include both OpenJDK and OpenJFX. Alternatively, you can simply include OpenJFX in any Java project by referencing the project in a Maven POM or Gradle build file.
This steady evolution of JavaFX, now OpenJFX, indicates it's the modern approach for GUI development with Java. Existing Swing applications that must be updated to a new layout with an up-to-date approach can be gradually migrated as both frameworks can coexist.
JavaFX example
The following code shows a simple JavaFX application written to be executed using JBang:
///usr/bin/env jbang "$0" "$@" ; exit $?
//DEPS org.openjfx:javafx-controls:23
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
/**
* You can execute this example with jbang.dev,
* which will fetch a matching Java runtime if needed:
* $ jbang HelloWorldJavaFX.java
*/
public class HelloWorldJavaFX extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Label label = new Label("This is a label");
root.getChildren().add(label);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("Hello JavaFX World!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
And here is what the code looks like when it runs in JBang:

Which to choose: Swing or JavaFX?
So now, which to choose for your next Java project: Java Swing or JavaFX?
Swing played an important role in the evolution of GUI development on the Java platform. Many legacy programs still use Swing components, which means interest in the JFC classes that support Swing will continue far into the future.
However, JavaFX represents the future of Java desktop user interface development because it offers more effective, flexible and visually compelling features.
For any greenfield projects that require Java GUI support, avoid Swing. JavaFX is the correct choice.
Frank Delporte is a Java Champion, software engineer, documentation writer and author who loves to write, podcast and experiment with Java and JavaFX.